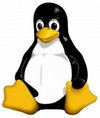
This has more applications than with just the chkconfig command. However, I found this script to be useful and wanted to add it to my database of useful scripts.
This script will chkconfig off the services listed in the services variable.
#!/bin/bash # chkconfig-services-off.sh # # Add services here services="vsftpd yum-updatesd vncserver sendmail" arr=$(echo $services | tr " " "\n") for x in $arr do /sbin/chkconfig $x off &>/dev/null || echo -e "\033[1m * Service $x not exists\033[0m"
By changing the off to on, the script will chkconfig on the services listed in the services variable.
#!/bin/bash # chkconfig-services-on.sh # # Add services here services="vsftpd yum-updatesd vncserver sendmail" arr=$(echo $services | tr " " "\n") for x in $arr do /sbin/chkconfig $x on &>/dev/null || echo -e "\033[1m * Service $x not exists\033[0m"
Here is the breakdown of one of the components of this script where I needed clarification. It turns out that those \033[1m and \033[0m are used for changing the default color of the text.
echo -e "\033[1m * Service $x not exists\033[0m"
This is a great writeup with additional colors.
Here is a list of colors: 30 = black 31 = red 32 = green 33 = yellow 34 = blue 35 = magenta 36 = cyan 37 = lightgray Now these are the basic colors. Usage of "3[": This is to handle the console cursor. * 'm' character at the end of each of the following sentences is used as a stop character, where the system should stop and parse the 3[ syntax. 3[0m - is the default color for the console 3[0;#m - is the color of the text, where # is one of the codes above 3[1m - makes text bold 3[1;#m - makes colored text bold** 3[2;#m - colors text according to # but darker 3[4;#m - colors text in # and underlines 3[7;#m - colors the background according to # 3[9;#m - colors text and strikes it 3[A - moves cursor one line above 3[B - moves cursor one line under 3[C - moves cursor one spacing to the right 3[D - moves cursor one spacing to the left 3[E - ? 3[F - ? 3[2K - erases everything written on line before this. **(this could be useful for those who want more colors. The effect given by bold will give a color change effect)
Examples
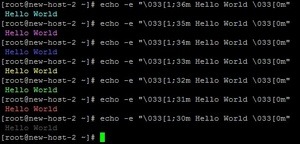
Source(s)
http://www.kborisov.com/2011/05/06/how-to-chkconfig-off-multiple-services/
http://www.cplusplus.com/forum/unices/36461/