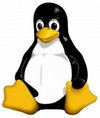
When using if then statements in your scripts, these statements will likely take up multiple lines. Sometimes, you may want a simple if then statement and would prefer to write only one line of code. This is a quick tip I picked up the other day. It is a simplification and explanation off how to combine multiple lines of code into a single line of code.
For example, this if then statement where if the file exists, then delete it.
if [ -f file ] then rm file fi
It can be expressed as
[ -f file ] && rm file
This statement is read, if command1 is true (has an exit status of zero) then perform command2.
The || construct will do the opposite:
command1 || command 2
If the exit status of command1 is false (non-zero), command2 will be executed.
UNIX pipelines may be used on either side of && or ||, and both constructs can be used on the same line to simulate the logic of an if-else statement. Consider the following lines of code:
if [ -f file ] then rm file else print "file was not found, or is not a regular file" fi
Using && and || together, this block of code can be reduced to:
[ -f file ] && rm file || print "file was not found, or is not a regular file"
Finally, multiple commands can be executed based on the result of command1 by incorporating braces and semi-colons:
command1 && { command2 ; command3 ; command4 ; }
If the exit status of command1 is true (zero), commands 2, 3, and 4 will be performed.
Other examples
[ -d /var/logs ] && echo "Directory exists" || echo "Directory does not exists" [ -d /dumper/fack ] && echo "Directory exists" || echo "Directory does not exists"
Source(s)
http://www.livefirelabs.com/unix_tip_trick_shell_script/june_2003/06232003.htm
https://www.cyberciti.biz/tips/find-out-if-file-exists-with-conditional-expressions.html